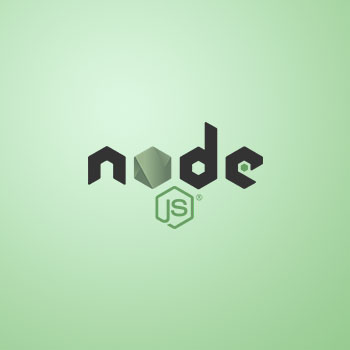
收录了这篇文章
常用的Node.js图片处理模块,有node-image、sharp和jimp。node-image很轻量,但是处理gif有点问题,更新还有点慢了。sharp跨操作系统部署的时,常常还需要编译一下。所以,jimp也是一个很好的选择。
介绍:
An image processing library for Node written entirely in JavaScript, with zero native dependencies.
看这个官方介绍也说了,完全使用Node写的的库,无原生依赖。 支持图片类型bmp、gif、jpeg、png和tiff。
安装:
npm install --save jimp
示例代码
Promise will never resolve if callback is passed
回调必须执行通过,否则规约将永远不会编程完成状态。
var Jimp = require('jimp'); // open a file called "lenna.png" Jimp.read('lenna.png', (err, lenna) => { if (err) throw err; lenna .resize(256, 256) // resize .quality(60) // set JPEG quality .greyscale() // set greyscale .write('lena-small-bw.jpg'); // save });
使用 Promises:
Jimp.read('lenna.png') .then(lenna => { return lenna .resize(256, 256) // resize .quality(60) // set JPEG quality .greyscale() // set greyscale .write('lena-small-bw.jpg'); // save }) .catch(err => { console.error(err); });
Jimp.read 的基础用法
The static Jimp.read method takes the path to a file, URL, dimensions, a Jimp instance or a buffer and returns a Promise:
静态函数 Jimp.read可以接受多种类型的参数,比如:文件、链接、Jimp实例或者一个缓存,返回一个Promise对象。
Jimp.read('./path/to/image.jpg') .then(image => { // Do stuff with the image. }) .catch(err => { // Handle an exception. }); Jimp.read('http://www.example.com/path/to/lenna.jpg') .then(image => { // Do stuff with the image. }) .catch(err => { // Handle an exception. }); Jimp.read(jimpInstance) .then(image => { // Do stuff with the image. }) .catch(err => { // Handle an exception. }); Jimp.read(buffer) .then(image => { // Do stuff with the image. }) .catch(err => { // Handle an exception. });
Methods
常用方法
Once the promise is fulfilled, the following methods can be called on the image:
/* Resize */ image.contain( w, h[, alignBits || mode, mode] ); // scale the image to the given width and height, some parts of the image may be letter boxed image.cover( w, h[, alignBits || mode, mode] ); // scale the image to the given width and height, some parts of the image may be clipped image.resize( w, h[, mode] ); // resize the image. Jimp.AUTO can be passed as one of the values. image.scale( f[, mode] ); // scale the image by the factor f image.scaleToFit( w, h[, mode] ); // scale the image to the largest size that fits inside the given width and height // An optional resize mode can be passed with all resize methods. /* Crop */ image.autocrop([tolerance, frames]); // automatically crop same-color borders from image (if any), frames must be a Boolean image.autocrop(options); // automatically crop same-color borders from image (if any), options may contain tolerance, cropOnlyFrames, cropSymmetric, leaveBorder image.crop( x, y, w, h ); // crop to the given region /* Composing */ image.blit( src, x, y, [srcx, srcy, srcw, srch] ); // blit the image with another Jimp image at x, y, optionally cropped. image.composite( src, x, y, [{ mode, opacitySource, opacityDest }] ); // composites another Jimp image over this image at x, y image.mask( src, x, y ); // masks the image with another Jimp image at x, y using average pixel value image.convolute( kernel ); // applies a convolution kernel matrix to the image or a region /* Flip and rotate */ image.flip( horz, vert ); // flip the image horizontally or vertically image.mirror( horz, vert ); // an alias for flip image.rotate( deg[, mode] ); // rotate the image clockwise by a number of degrees. Optionally, a resize mode can be passed. If `false` is passed as the second parameter, the image width and height will not be resized. /* Colour */ image.brightness( val ); // adjust the brighness by a value -1 to +1 image.contrast( val ); // adjust the contrast by a value -1 to +1 image.dither565(); // ordered dithering of the image and reduce color space to 16-bits (RGB565) image.greyscale(); // remove colour from the image image.invert(); // invert the image colours image.normalize(); // normalize the channels in an image /* Alpha channel */ image.hasAlpha(); // determines if an image contains opaque pixels image.fade( f ); // an alternative to opacity, fades the image by a factor 0 - 1. 0 will haven no effect. 1 will turn the image image.opacity( f ); // multiply the alpha channel by each pixel by the factor f, 0 - 1 image.opaque(); // set the alpha channel on every pixel to fully opaque image.background( hex ); // set the default new pixel colour (e.g. 0xFFFFFFFF or 0x00000000) for by some operations (e.g. image.contain and /* Blurs */ image.gaussian( r ); // Gaussian blur the image by r pixels (VERY slow) image.blur( r ); // fast blur the image by r pixels /* Effects */ image.posterize( n ); // apply a posterization effect with n level image.sepia(); // apply a sepia wash to the image image.pixelate( size[, x, y, w, h ]); // apply a pixelation effect to the image or a region /* 3D */ image.displace( map, offset ); // displaces the image pixels based on the provided displacement map. Useful for making stereoscopic 3D images.
Resize modes
图片缩放的模式
The default resizing algorithm uses a bilinear method as follows:
image.resize(250, 250); // resize the image to 250 x 250 image.resize(Jimp.AUTO, 250); // resize the height to 250 and scale the width accordingly image.resize(250, Jimp.AUTO); // resize the width to 250 and scale the height accordingly
项目地址:
https://github.com/oliver-moran/jimp/tree/master/packages/jimp